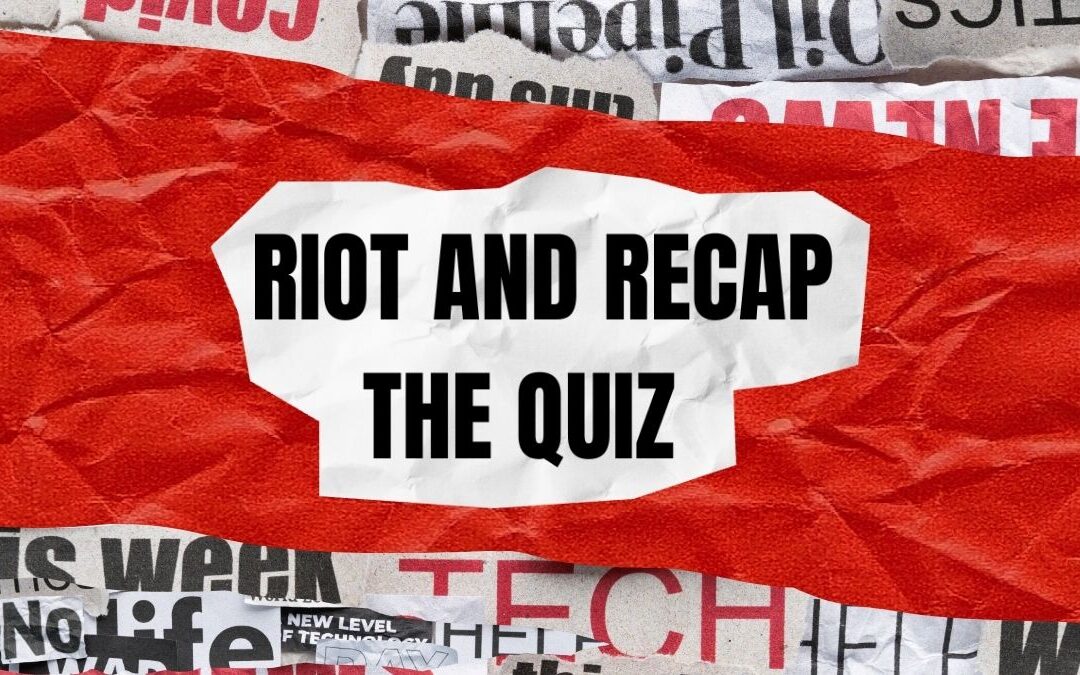
RIOT AND RECAP: THE QUIZ
Welcome to the latest edition of Riot and Recap.
It’s been another week of joy here in batsh*it bonkers Britain, so why not relive it all and test your knowledge?
Grab a brew (with tap water, while you still can) and wade through the chaos – and sewage – with us.
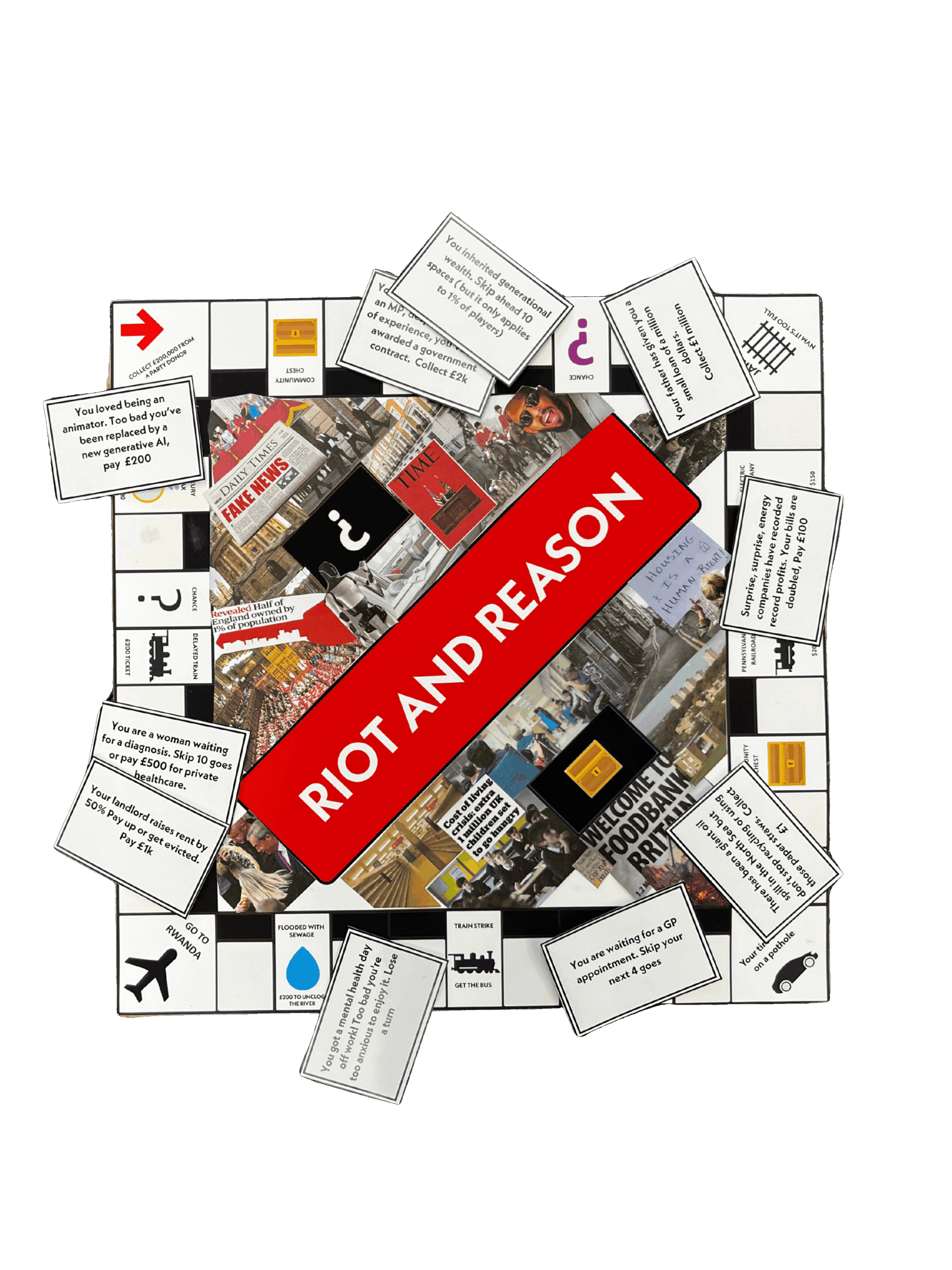
Inequality isn’t a glitch in this rigged game of life – it’s the design. One step foward feels like 10 shoves back. When everyone’s dealt a different hand, don’t just be mad at the players, but the system.
Keep an eye out for the Chance Cards across our site – a reminder of the broken rules in this rat race.
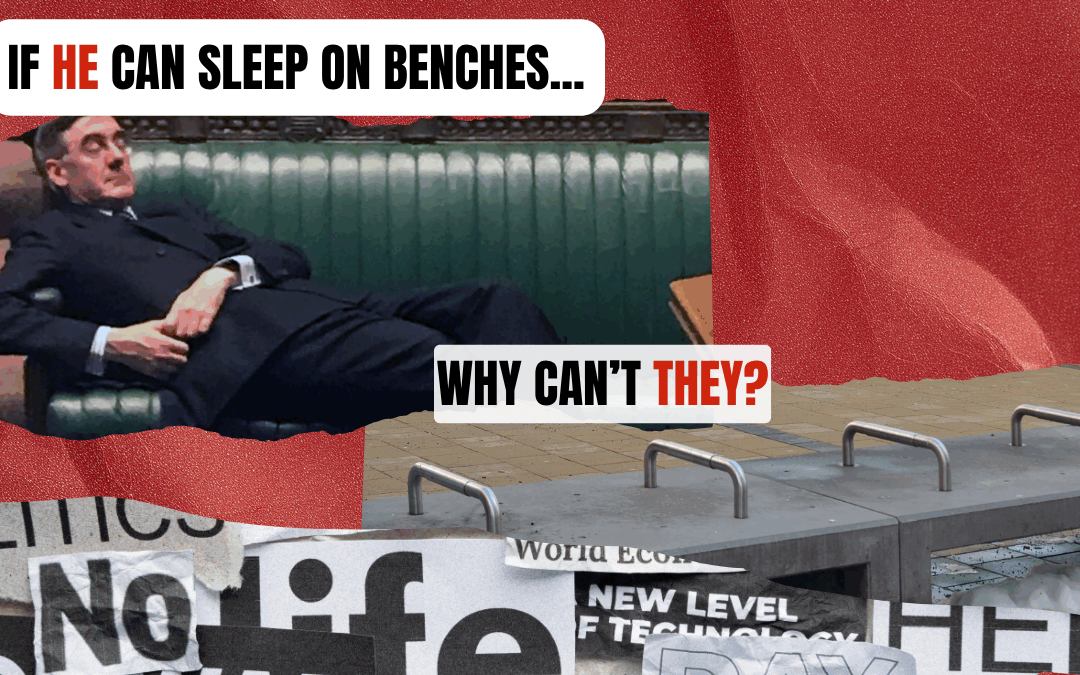
Concrete Cruelty: How hostile architecture designs people out of cities
Jacob Rees-Mogg asleep on bench in house of commons. Hostile architecture bench with metal dividers Hostile architecture: The bench with a metal divider, the metal spikes poking through the concrete, the slanted roof of the shelter that lets the rain in. These aren’t...
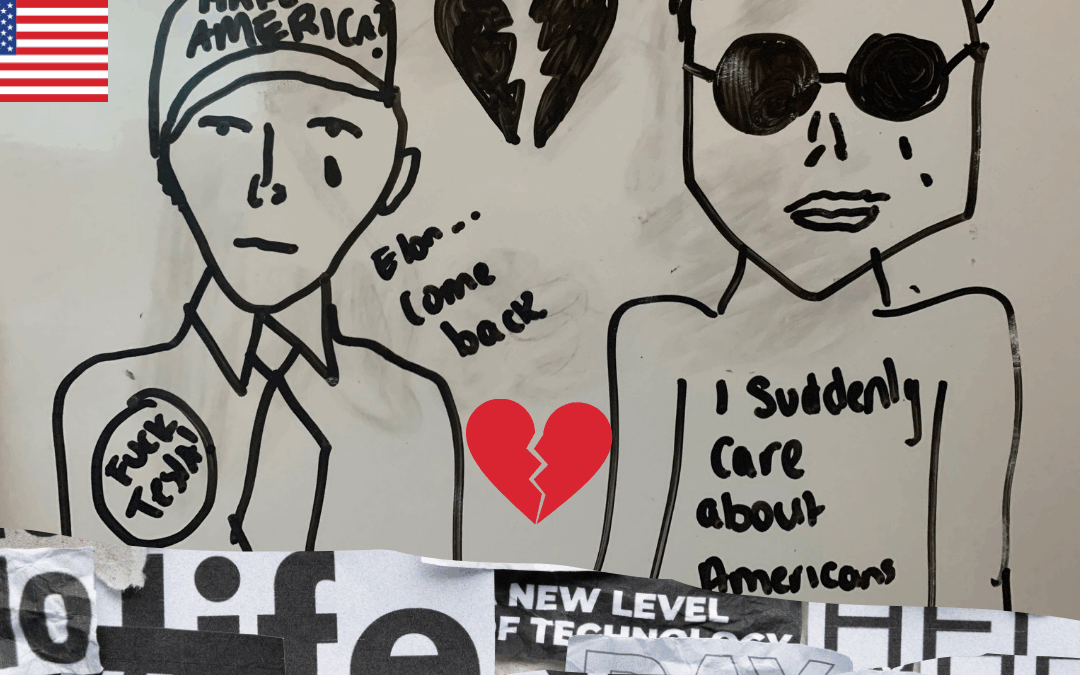
THE FIGHT HOUSE: TRUMP DUMPED BY ELON
There’s a Taylor Swift song out there pending, because a bromance has truly crumbled. It’s not just breaking news dear readers, it’s breaking our beaten hearts. Perhaps the line up of Love Island being released was too tempting for the two.
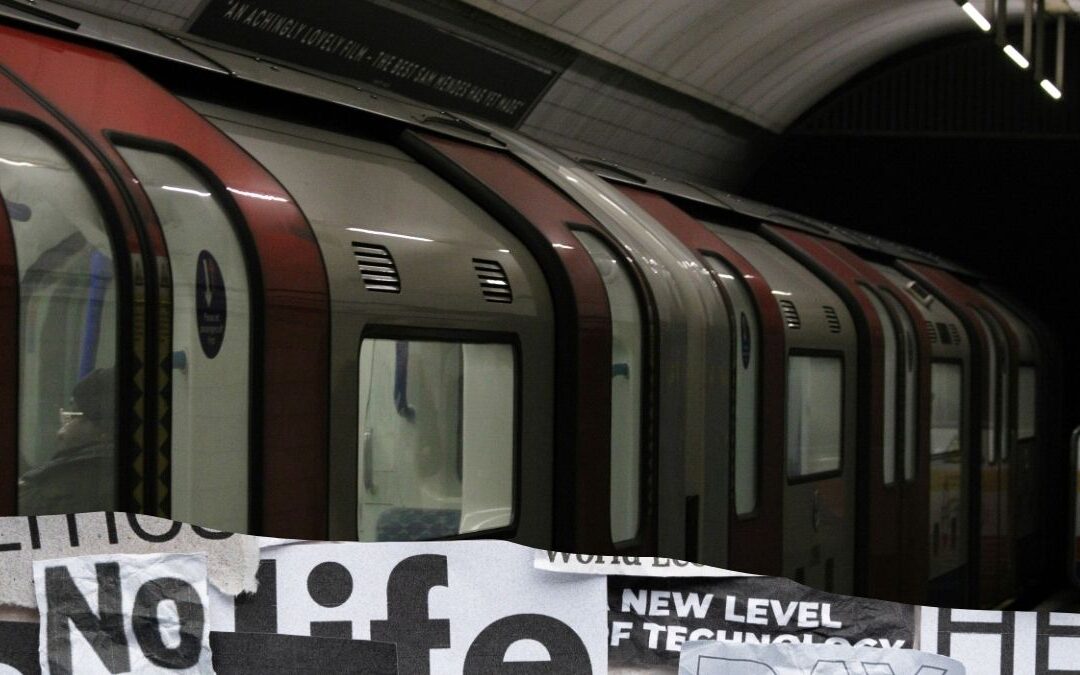
All Aboard (Maybe): Labour’s £15.6bn Transport Plan Hits the Rails
You know things are bad when Britain’s trains become a political love language. Delayed, overcrowded and eye-wateringly expensive. Our transport system is a national joke without a punchline, but this week, Labour Chancellor Rachel Reeves tried to flip the...
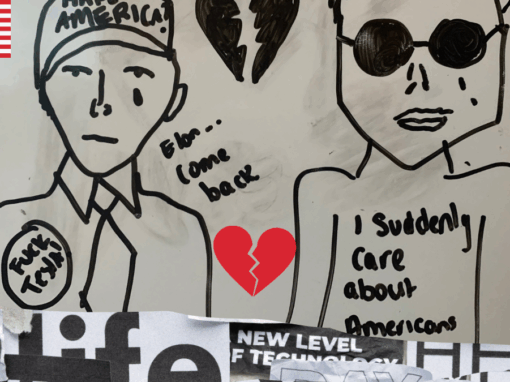
THE FIGHT HOUSE: TRUMP DUMPED BY ELON
My incredible photo of Elon and Trump There's a Taylor Swift song out there pending, because a bromance has truly crumbled. It's not just breaking news dear readers, it's breaking our beaten hearts. Perhaps the line up of Love Island being released was too tempting...
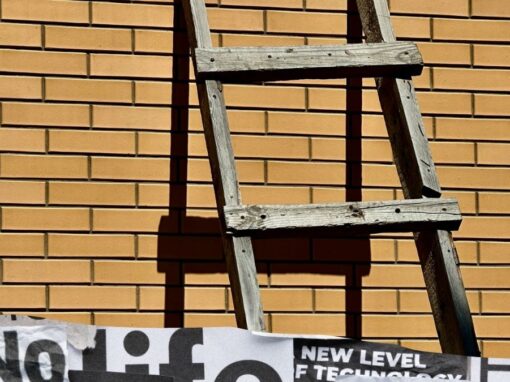
Why the property ladder feels like more snakes than ladders for young brits
If you’re under 35 and haven’t bought a house yet, you’ve probably felt the weight of it. Not just the financial pressure, but also the cultural expectations. The nagging sense that you’re falling behind an invisible adulting schedule. Your parents did it, your boss...
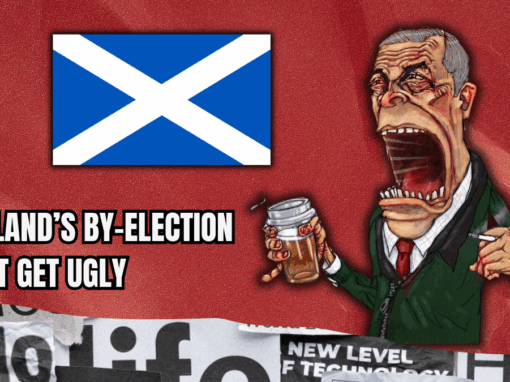
When the system cracks, Reform UK might just slip in
As voters in the Hamilton constituency heads to the polls, a once-fringe party is creeping into the mainstream. Reform UK’s surge in Scotland signals more than voter frustration - it’s a red flag waving in plain sight. Behind the anti-migrant soundbites and “ordinary...
Culture And Chaos
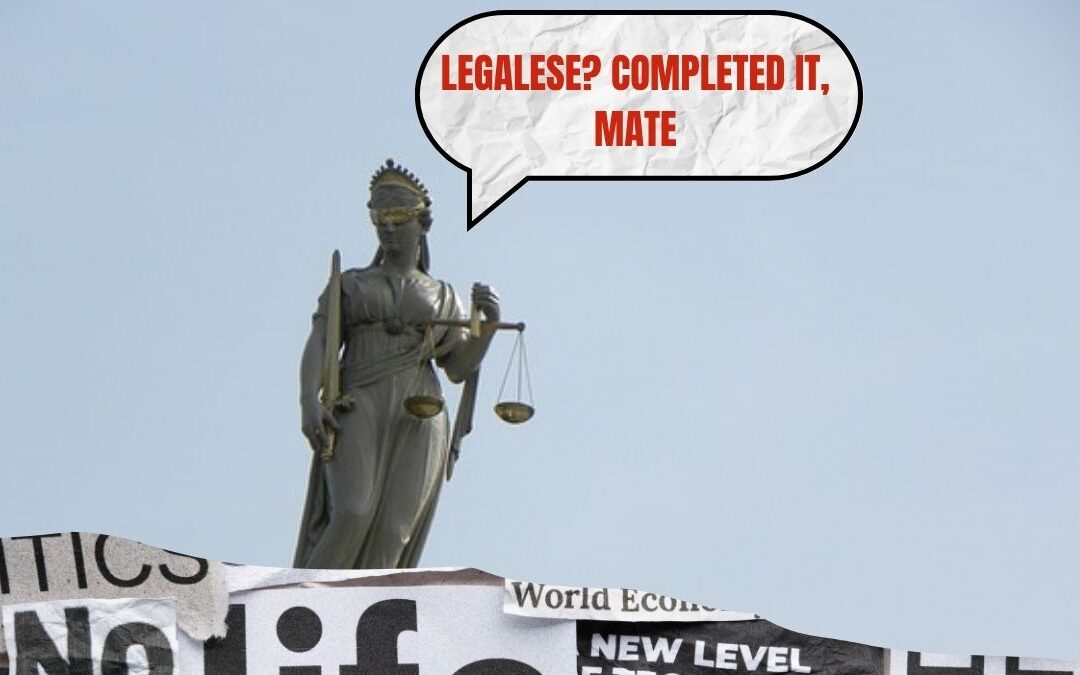
Objection: legal jargon is injustice
Ignorantia Legis Neminem Excusat. A foundational principle of law. For normal people who are yet to have their Elle Woods moment, or casually take up Latin, this legal phrase means ignorance of the law excuses no one. Ironic. Because if the law is meant to uphold...
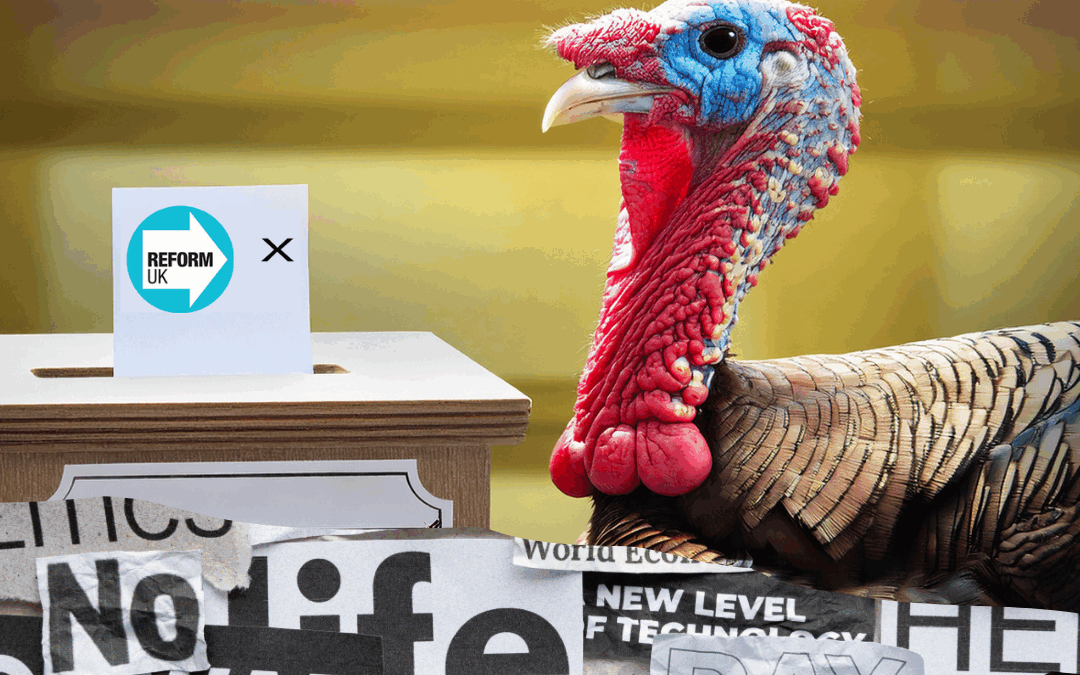
A turkey voting for Christmas? The unexpected Reform Voters
Reform’s message is finding support in unexpected places — including among some Hong Kong immigrants in the UK. Is it difficult to imagine someone fleeing to the UK due to political turmoil, supporting a party that is calling for stricter immigration controls? This...
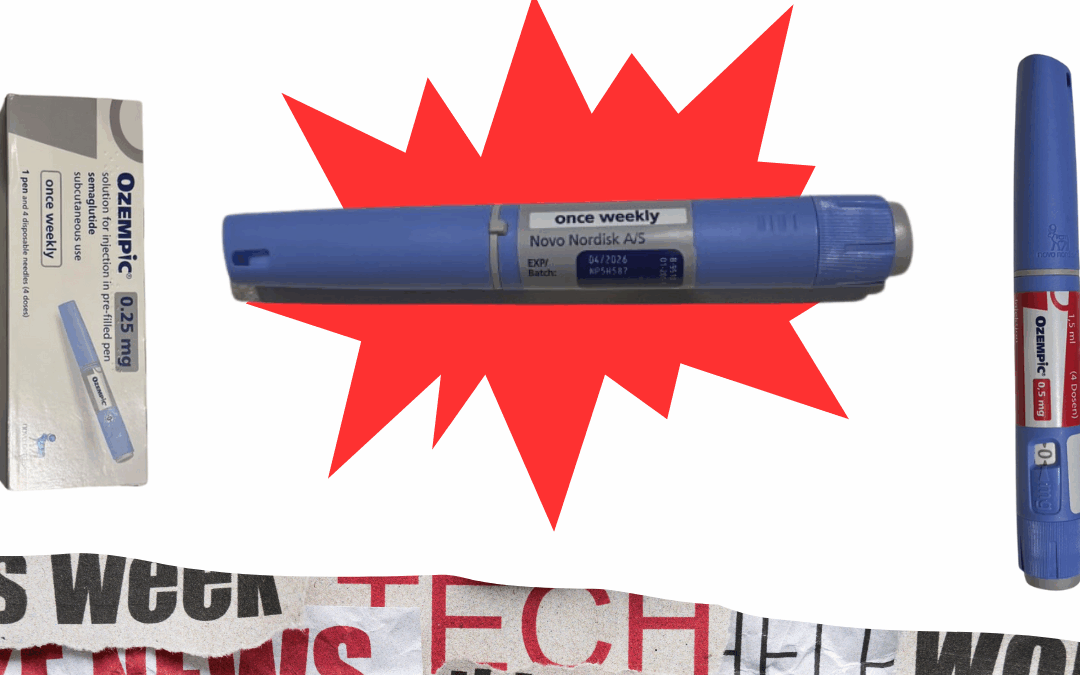
Mission Slimpossible: The Weight of Secrecy
One in ten women are currently taking weight loss injections, but how many would tell you they are? GLP-1 medications have been viciously stigmatised and criticised online. We’ve spoken to those jabbing for joy and those who are secretly using them. If needles make you squeamish, I suggest you look away…
Unfiltered Voices
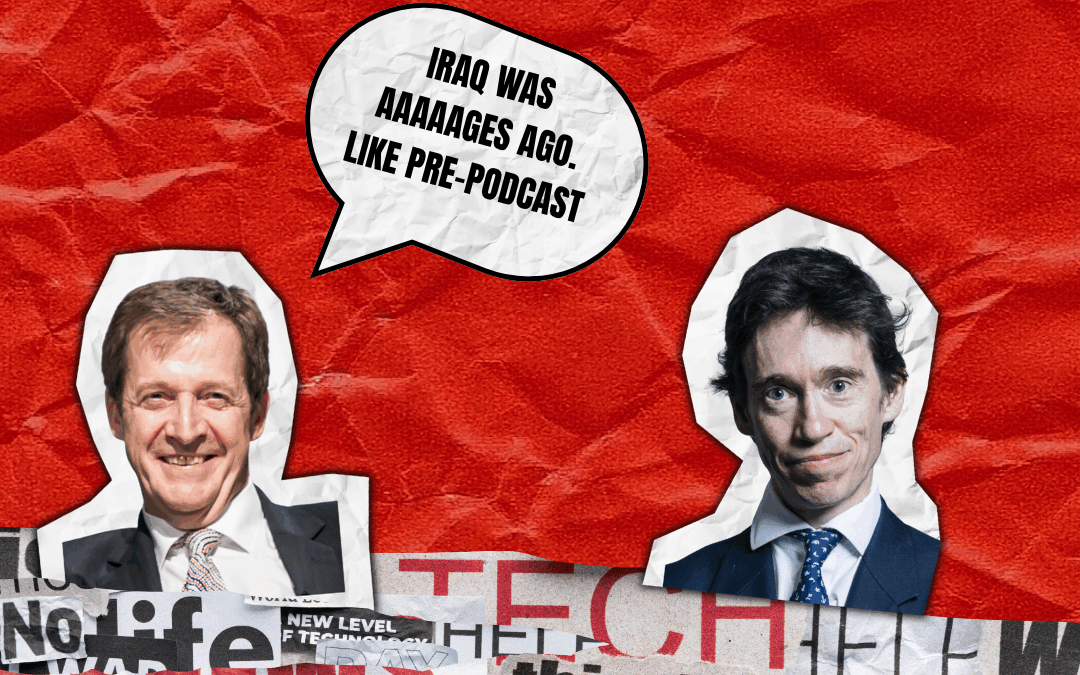
The Rest is Politics : It’s not all ancient histories
It's one of the nation's favourite political podcasts but the messy legacies of it's hosts make it hard to love. With 1.2 million monthly views and millions of listeners ‘The Rest is Politics’ has proven to be one of the UK’s most popular podcasts but as I find myself...
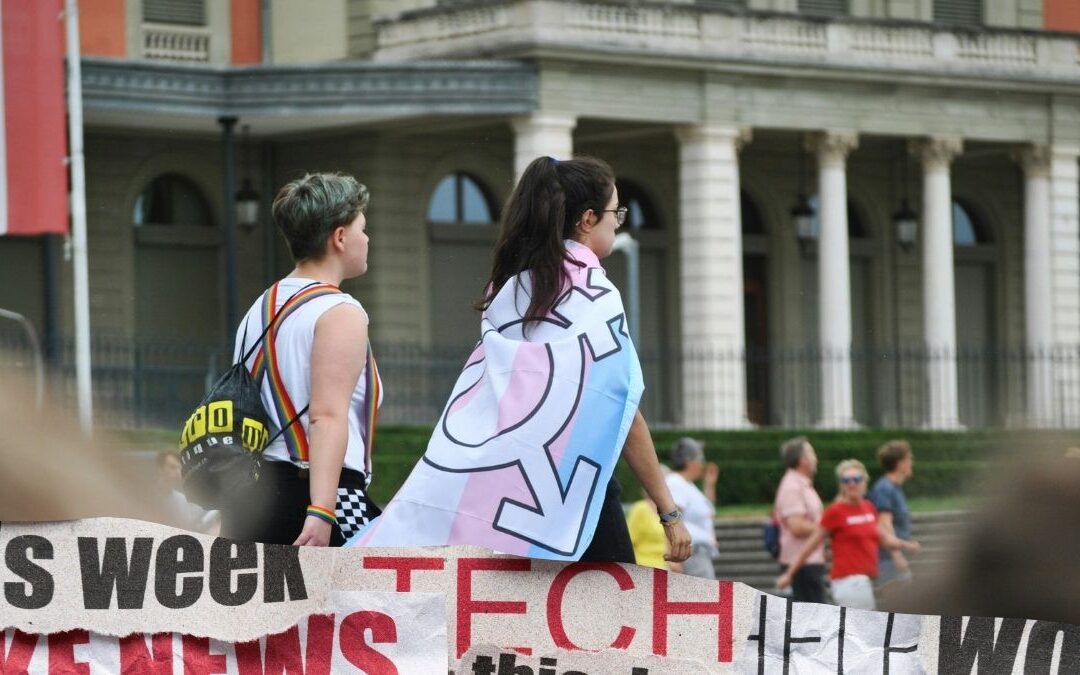
UK trans rights under attack: Not the threat, but the target
Trans people are not the problem and they are not a threat, but they are being threatened, but you wouldn’t know that from looking at headlines, right now we’re watching a full blown moral panic unfold in real time. Across the UK a number of political attacks are...
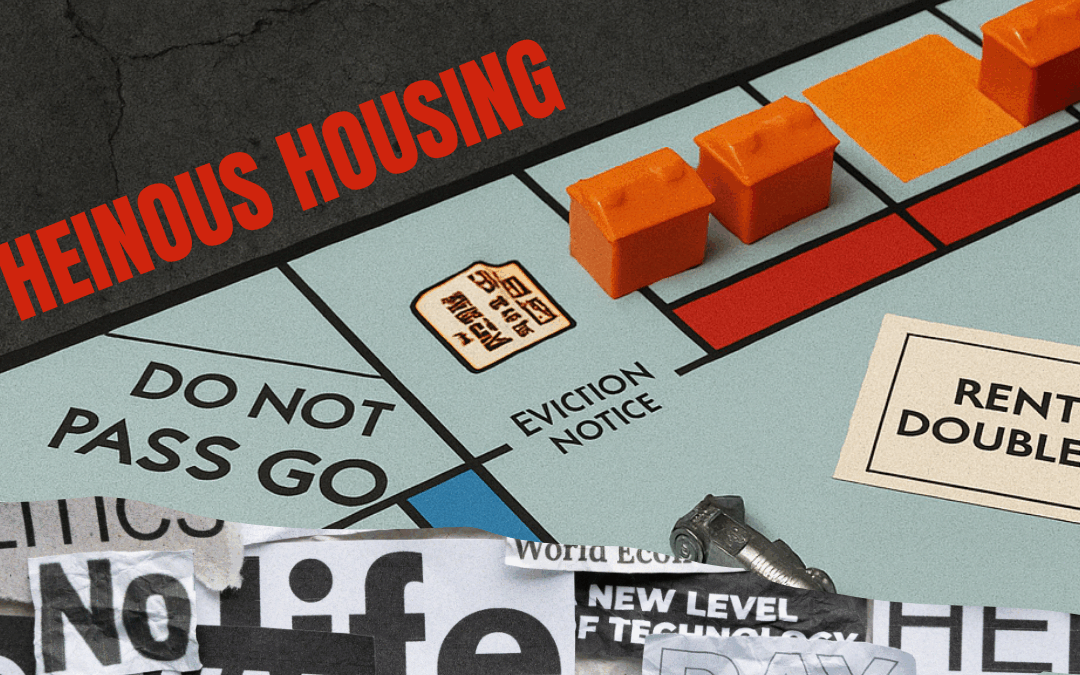
Heinous Housing
Let’s not sugar-coat it: Housing in the UK in 2025 feels dystopian. Like you’re competing in a brutal hunger games reboot. Except the price is rocket high rent prices that will have you living pay check to pay check and expect you to be grateful. If you’re aged 18-35,...
What Now?
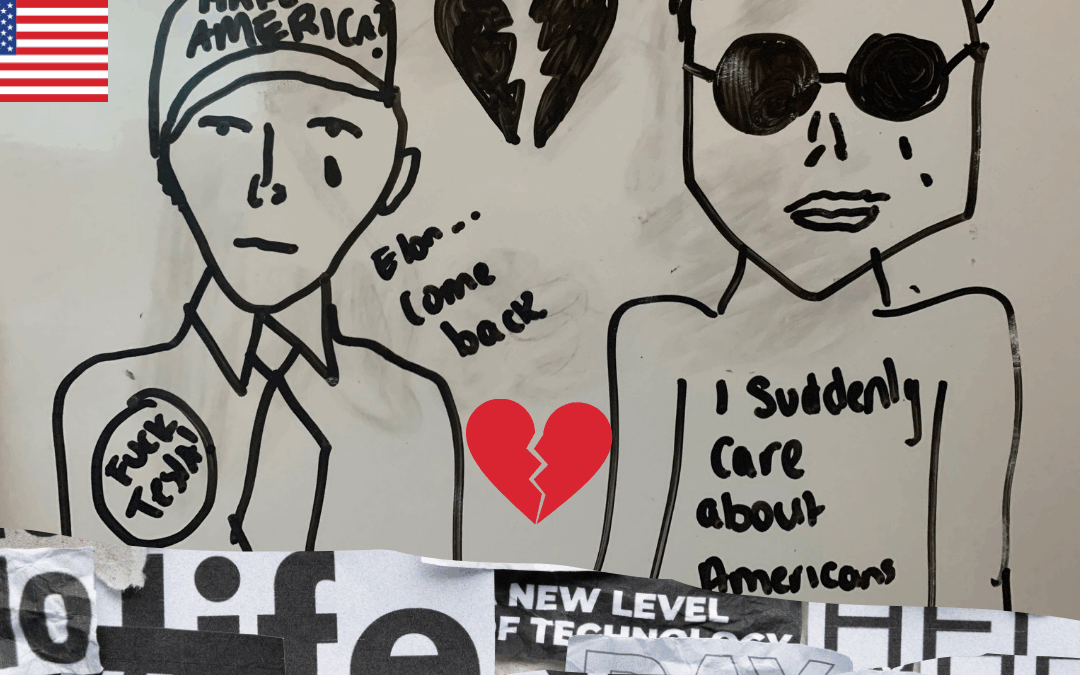
THE FIGHT HOUSE: TRUMP DUMPED BY ELON
There’s a Taylor Swift song out there pending, because a bromance has truly crumbled. It’s not just breaking news dear readers, it’s breaking our beaten hearts. Perhaps the line up of Love Island being released was too tempting for the two.
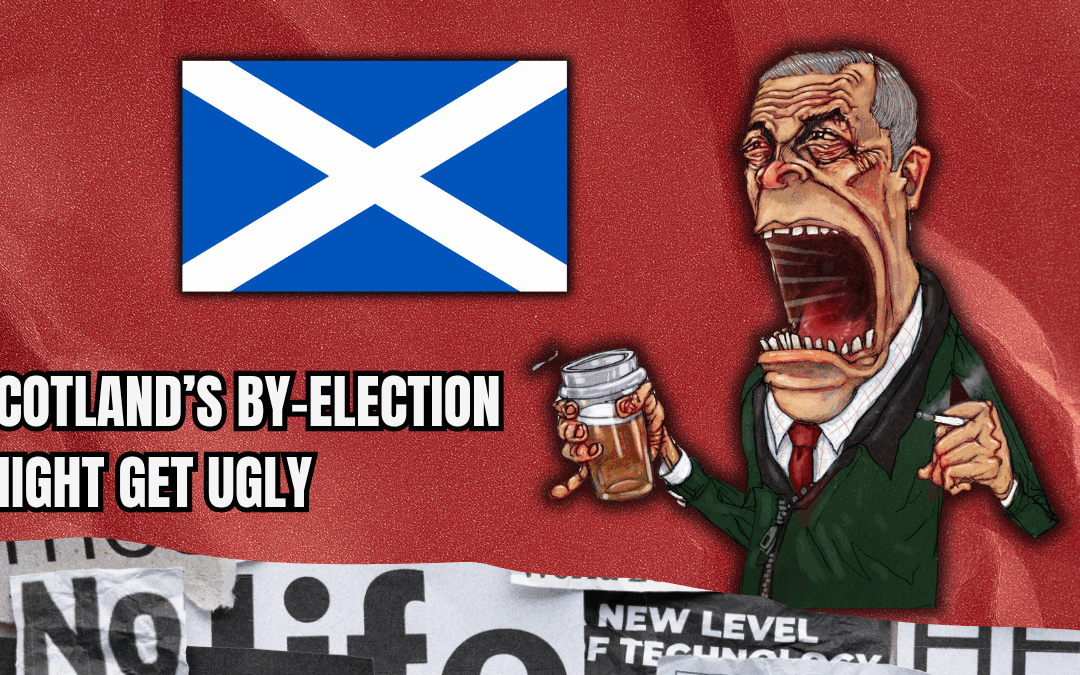
When the system cracks, Reform UK might just slip in
As voters in the Hamilton constituency heads to the polls, a once-fringe party is creeping into the mainstream. Reform UK’s surge in Scotland signals more than voter frustration - it’s a red flag waving in plain sight. Behind the anti-migrant soundbites and “ordinary...
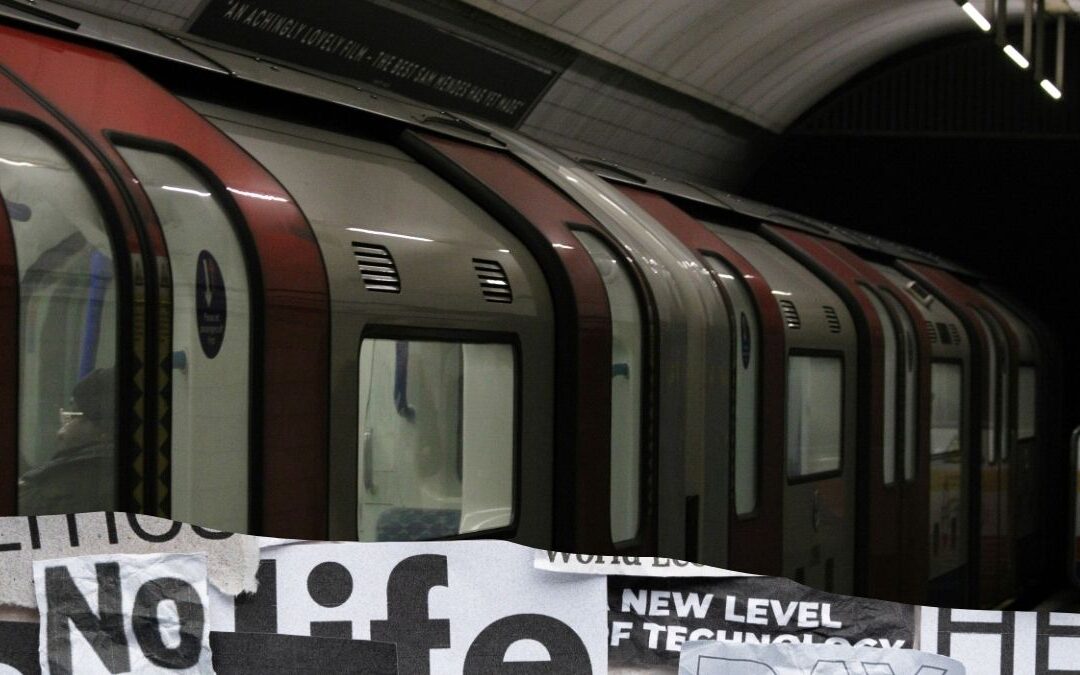
All Aboard (Maybe): Labour’s £15.6bn Transport Plan Hits the Rails
You know things are bad when Britain’s trains become a political love language. Delayed, overcrowded and eye-wateringly expensive. Our transport system is a national joke without a punchline, but this week, Labour Chancellor Rachel Reeves tried to flip the...
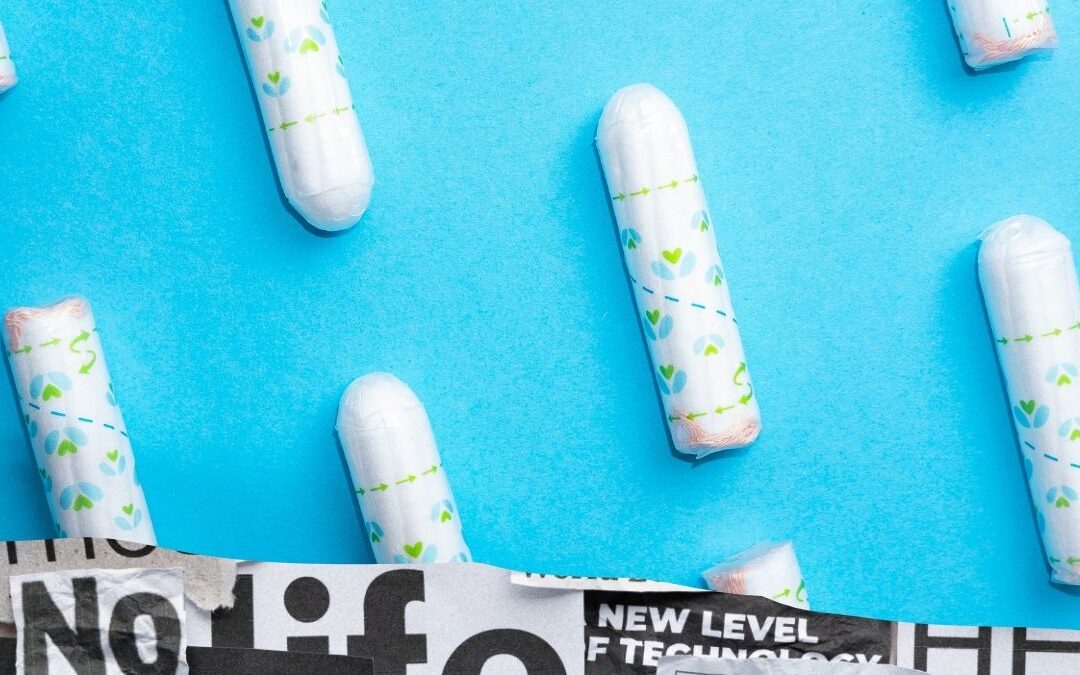
Toxic tampons: Pesticides found in UK period products
You’d think that in 2025, basic period care wouldn’t be contaminated with toxic chemicals, but welcome to late stage capitalism where even bleeding safely has a price tag. A new report from PAN UK, the Women’s Environmental Network and the Pesticide collaboration just...
Public Sh*t Show
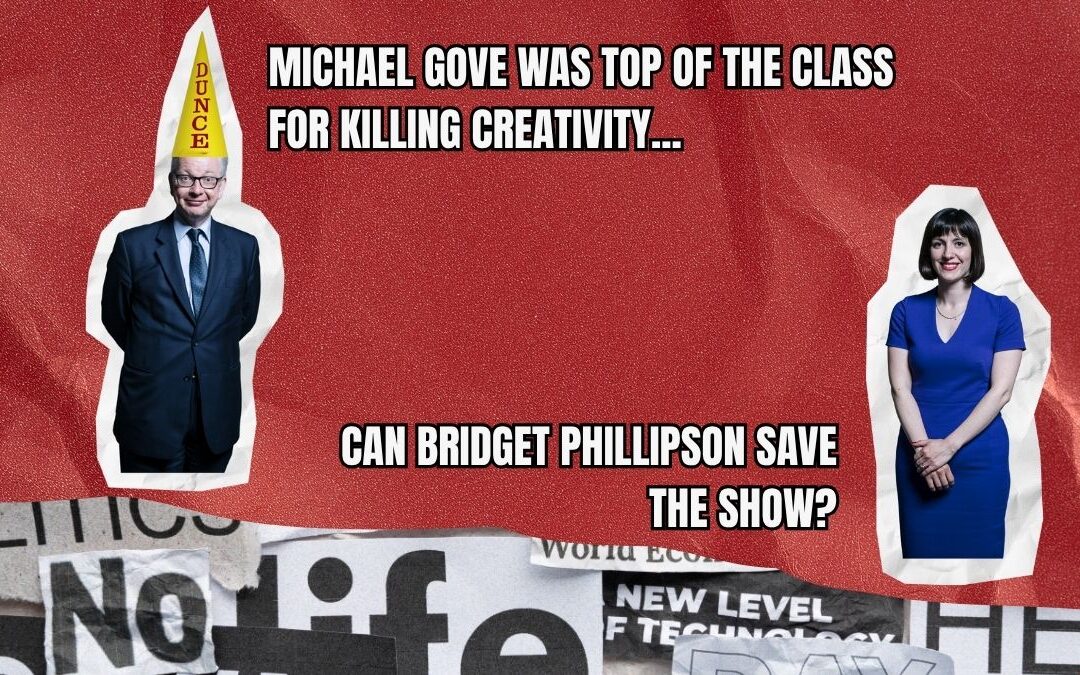
Spreadsheets and silence: the classist curriculum
We need to stop pretending. Fixing our inherently unequal education system is never going to be done. But narrowing opportunities and killing creativity in state schools is not helping solve the unbalanced equation given to children. Despite claims from the Tories...
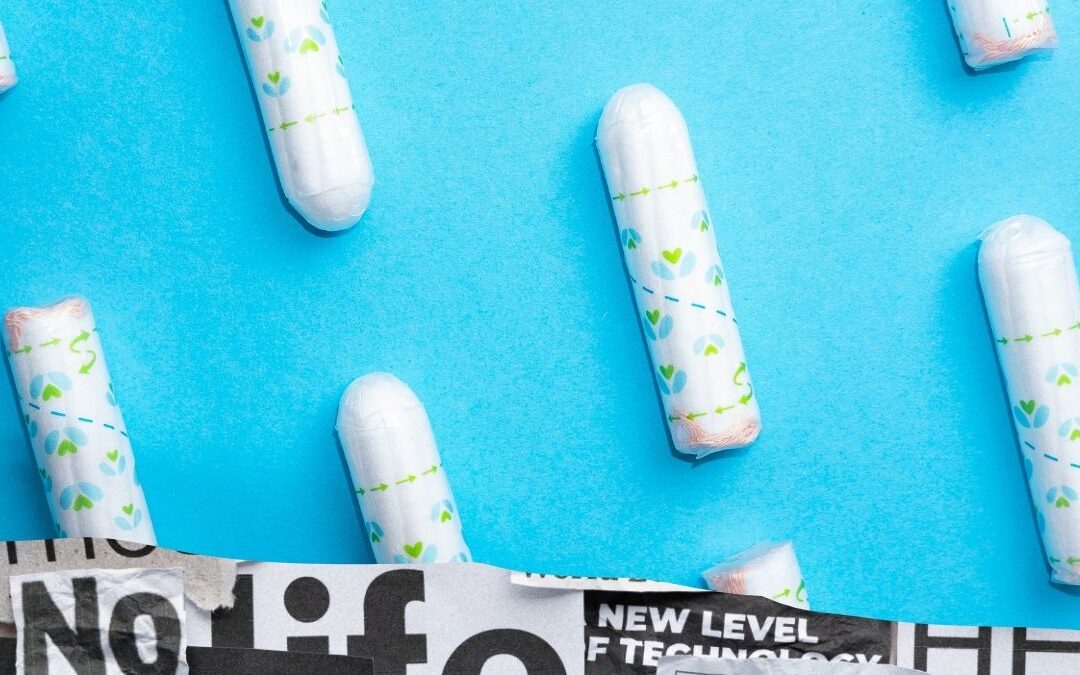
Toxic tampons: Pesticides found in UK period products
You’d think that in 2025, basic period care wouldn’t be contaminated with toxic chemicals, but welcome to late stage capitalism where even bleeding safely has a price tag. A new report from PAN UK, the Women’s Environmental Network and the Pesticide collaboration just...
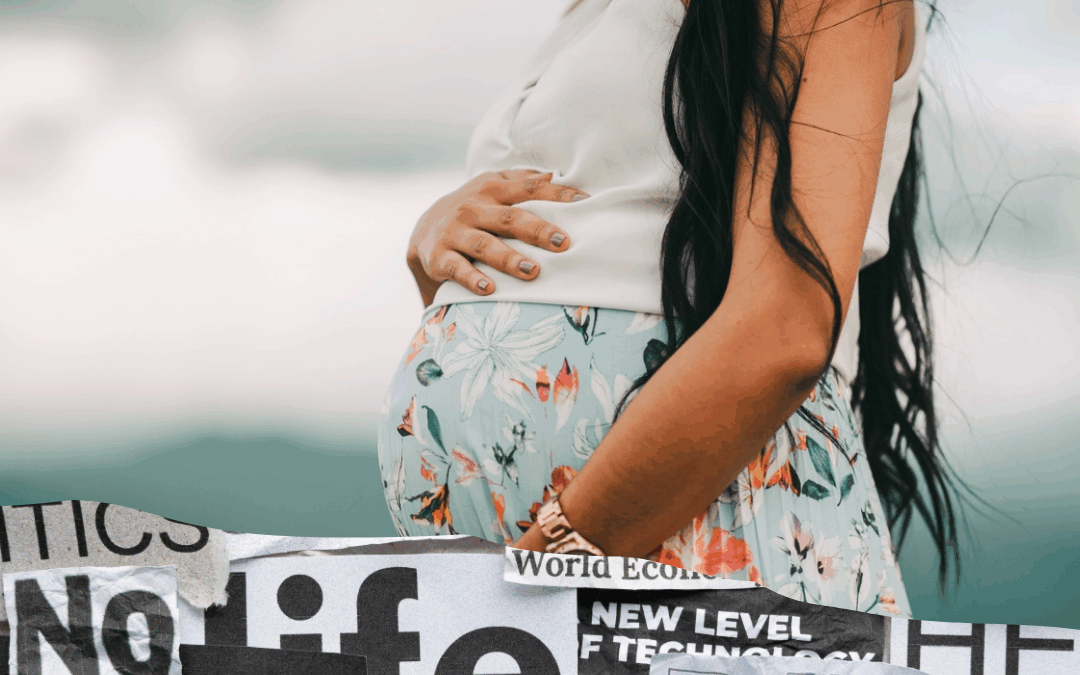
‘Surrogacy Slaves’ – How Georgia is obstructing women’s rights
At least one hundred Thai women are currently being held in Georgia by Chinese gang members, in a Handmaid’s Tale-esque limbo. Their passports were confiscated, and a fee has been imposed on their freedom. Like cooped chickens, they are being exploited for their human...
Tech-ing the P*ss
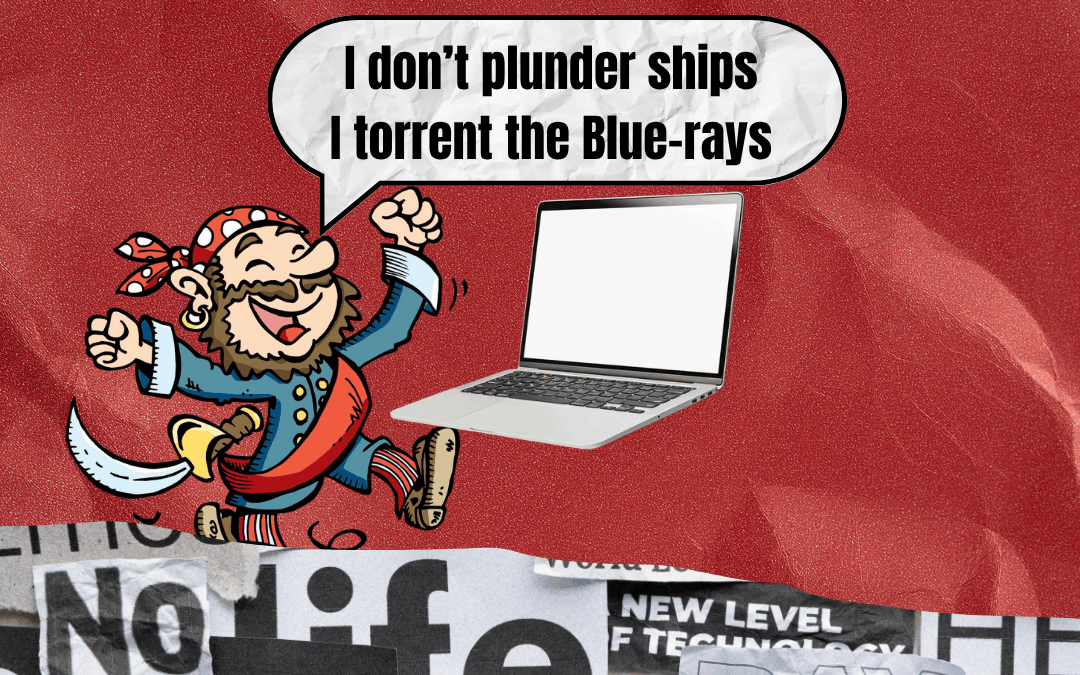
Hey Ho, It’s a Pirates life for me: Piracy and preservation
As media vanishes behind paywalls , digital piracy step in, not just to steal but preserve what corporations would rather let it sink Before your teacher slapped Narnia for the 5th time in the film club in year three, you were treated to another masterpiece of film...
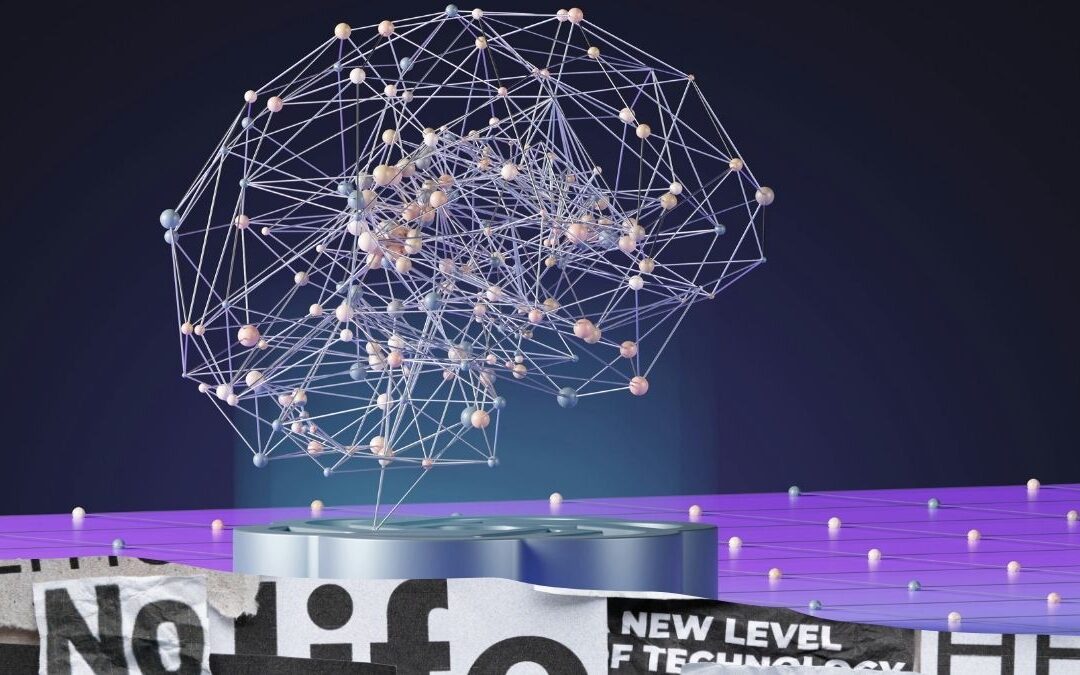
Your brain on AI: How algorithms are quietly rewiring the way you think
In 2025, artificial intelligence isn’t just assisting us, it’s subtly reshaping how we think, decide and even feel. Artificial intelligence isn’t coming for your job, it’s coming for your brain. Every time you ask ChatGPT to write you a cover letter, let TikTok...
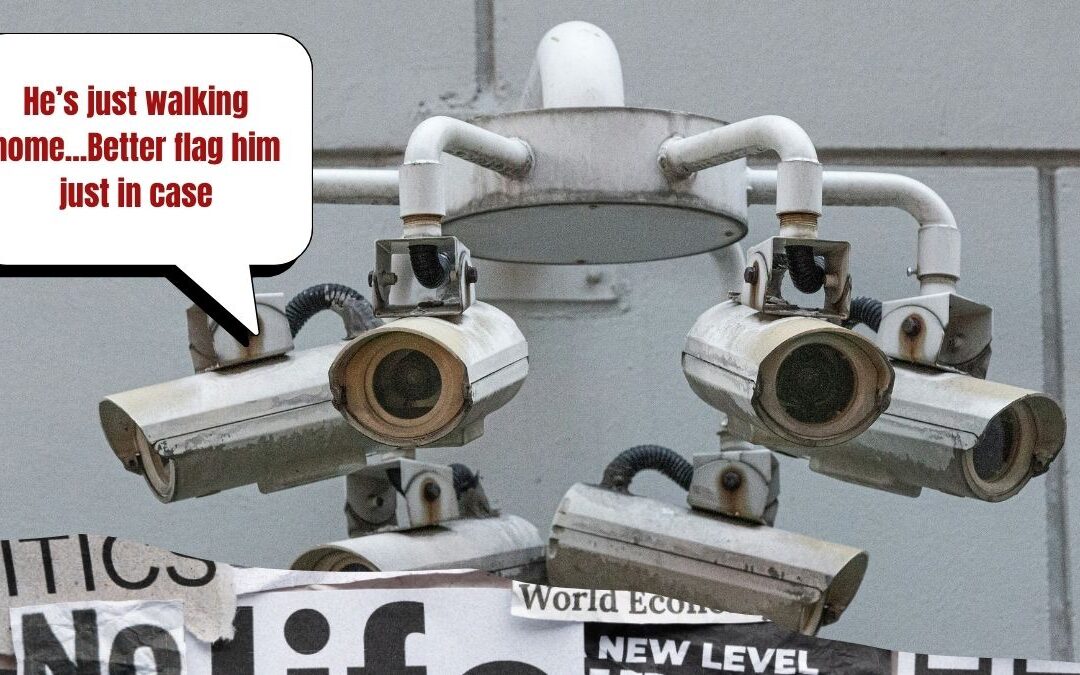
Face the Bias: How facial recognition fails people of colour
Facial recognition technology is the new thing of the future, you see it everywhere, when you unlock your phone, in the airport it’s like a friend that is here to stay. While it is built to detect faces, it somehow struggles with the basic concept that not all brown...